java.util 패키지는 개발에서 자주 사용되는 자료구조이며 날짜 정보를 제공해주는 유용한 API를 포함하고 있다.
이번 포스팅은 이 API에 대해서 알아보자.
대표적으로 Date 클래스와 Calendar 클래스가 있다.
- Date 클래스 : 특정 시점의 날짜를 표현하는 클래스, Date 객체 안에는 특정 시점의 연도, 월, 일, 시간 정보가 저장됨
- Calendar 클래스 : 달력을 표현한 클래스, 해당 운영체제의 연도, 월, 일, 요일, 오전/오후, 시간 등의 정보가 저장됨
< Date 클래스 >
Date 클래스는 날짜를 표현하는 클래스로 객체 간에 날짜 정보를 주고받을 대 매개 변수나 리턴 타입으로 주로 사용된다.
현재 시각의 Date 객체는 다음과 같이 생성할 수 있다.
Date now = new Date();
Date 객체의 toString() 메소드는 영문으로 된 날짜를 리턴하기 대문에 원하는 날짜 형식의 문자열을 얻고 싶다면 java.text 패키지의 SimpleDateFormat 클래스와 함께 사용하는 것이 좋다.,
즉, 원하는 형식 문자열로 다음과 같이 생성할 수 있다.
SimpleDateFormat sdf = new SimpleDateFormat("yyyy년 MM월 dd일 hh시 mm분 ss초");
SimpleDateFormat 객체를 얻었다면, format() 메소드를 호출해서 원하는 형식의 날짜 정보를 얻을 수 있다.
다음 코드는 현재 날짜를 출력하는 예제이다.
import java.text.*;
import java.util.*;
public class DateExample {
public static void main(String[] args) {
Date now = new Date();
String strNow1 = now.toString();
System.out.println(strNow1);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy년 MM월 dd일 hh시 mm분 ss초");
String strNow2 = sdf.format(now);
System.out.println(strNow2);
}
}
결과 :
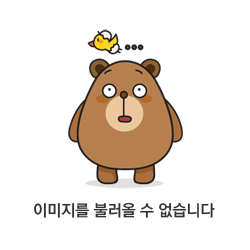
< Calendar 클래스 >
Calendar 클래스는 달력을 표현한 클래스이며 추상 클래스이다.
때문에 new 연산자를 사용해서 인스턴스를 생성할 수 없다.
Calendar 클래스의 정적 메소드인 getInstance() 메소드를 이용해서 현재 운영체제에 설정되어 있는 시간대를 기준으로 한 Calendar 하위 객체를 얻어올 수 있다.
Calender now = Calendar.getInstance();
Calendar 객체를 얻었다면 get() 메소드를 이용해서 날짜와 시간에 대한 정보를 읽을 수 있다.
int year = now.get(Calendar.YEAR); //연도를 리턴
int month = now.get(Calendar.MONTH)+1; //월을 리턴
int day = now.get(Calendar.DAY_OF_MONTH); //일을 리턴
int week = now.get(Calendar.DAY_OF_WEEK); //요일을 리턴(일 : 1, 월: 2, 화: 3, 수: 4, 목: 5, 금: 6, 토: 7)
int amPm = now.get(Calendar.AM_PM); //오전/오후를 리턴(오전: 0, 오후: 1)
int hour = now.get(Calendar.HOUR); //시를 리턴
int minute = now.get(Calendar.MINUTE); //분을 리턴
int second = now.get(Calendar.SECOND); //초를 리턴
get() 메소드를 호출할 때 사용한 매개값은 모두 Calendar 클래스에 선언되어 있는 상수들이다.
다음은 운영체제의 시간대를 기준으로 Calendar 얻는 예제이다.
import java.util.*;
public class CalendarExample {
public static void main(String[] args) {
Calendar now = Calendar.getInstance();
int year = now.get(Calendar.YEAR);
int month = now.get(Calendar.MONTH) + 1;
int day = now.get(Calendar.DAY_OF_MONTH);
int week = now.get(Calendar.DAY_OF_WEEK);
String strWeek = null;
switch(week) {
case Calendar.MONDAY:
strWeek = "월";
break;
case Calendar.TUESDAY:
strWeek = "화";
break;
case Calendar.WEDNESDAY:
strWeek = "수";
break;
case Calendar.THURSDAY:
strWeek = "목";
break;
case Calendar.FRIDAY:
strWeek = "금";
break;
case Calendar.SATURDAY:
strWeek = "토";
break;
default:
strWeek = "일";
}
int amPm = now.get(Calendar.AM_PM);
String strAmPm = null;
if(amPm == Calendar.AM) {
strAmPm = "오전";
} else {
strAmPm = "오후";
}
int hour = now.get(Calendar.HOUR);
int minute = now.get(Calendar.MINUTE);
int second = now.get(Calendar.SECOND);
System.out.print(year + "년 ");
System.out.print(month + "월 ");
System.out.println(day + "일 ");
System.out.print(strWeek + "요일 ");
System.out.println(strAmPm + " ");
System.out.print(hour + "시 ");
System.out.print(minute + "분 ");
System.out.println(second + "초 ");
}
}
결과 :
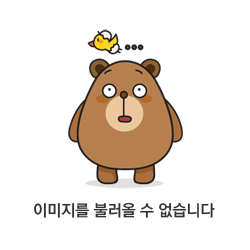
'프로그래밍언어 > Java' 카테고리의 다른 글
11-1 java.lang 패키지(2) (0) | 2022.03.02 |
---|---|
11-1 java.lang 패키지(1) (0) | 2022.02.23 |
10-2 예외 처리 (0) | 2021.12.27 |
10-1 예외 클래스 (0) | 2021.12.27 |
09-2 익명 객체 (0) | 2021.12.14 |